[C#/WPF/.NET8] 크롬 브라우저의 활성탭 웹 페이지에서 텍스트 추출하기 2
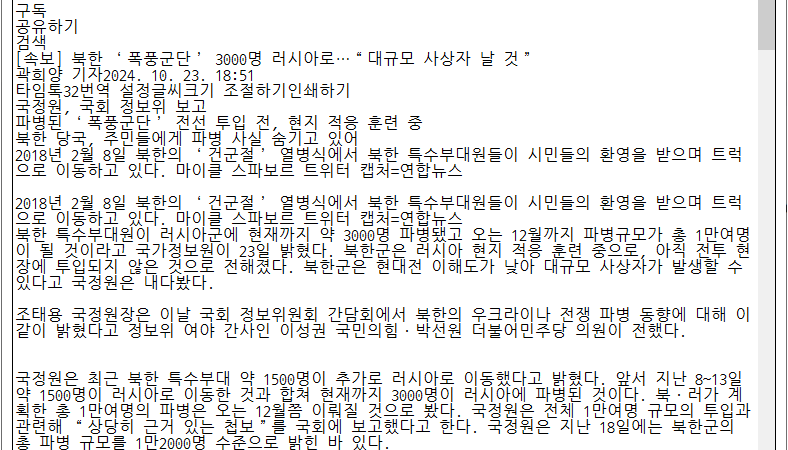
■ 크롬 브라우저의 활성탭 웹 페이지에서 텍스트를 추출하는 방법을 보여준다. ▶ ChromBrowserProcessHelper.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 |
using System; using System.Collections.Generic; using System.Diagnostics; using System.Runtime.InteropServices; using System.Text; using System.Threading; using System.Windows; namespace TestProject; /// <summary> /// 크롬 브라우저 프로세스 헬퍼 /// </summary> public class ChromBrowserProcessHelper { //////////////////////////////////////////////////////////////////////////////////////////////////// Structure ////////////////////////////////////////////////////////////////////////////////////////// Private #region 사각형 - RECT /// <summary> /// 사각형 /// </summary> [StructLayout(LayoutKind.Sequential)] private struct RECT { //////////////////////////////////////////////////////////////////////////////////////////////////// Field ////////////////////////////////////////////////////////////////////////////////////////// Public #region Field /// <summary> /// 왼쪽 /// </summary> public int Left; /// <summary> /// 위쪽 /// </summary> public int Top; /// <summary> /// 오른쪽 /// </summary> public int Right; /// <summary> /// 아래쪽 /// </summary> public int Bottom; #endregion } #endregion //////////////////////////////////////////////////////////////////////////////////////////////////// Delegate ////////////////////////////////////////////////////////////////////////////////////////// Public #region 윈도우 나열하기 대리자 - EnumerateWindowDelegate(windowHandle, longParameter) /// <summary> /// 윈도우 나열하기 대리자 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <param name="longParameter">LONG 매개 변수</param> /// <returns>처리 결과</returns> public delegate bool EnumerateWindowDelegate(IntPtr windowHandle, IntPtr longParameter); #endregion //////////////////////////////////////////////////////////////////////////////////////////////////// Import ////////////////////////////////////////////////////////////////////////////////////////// Static //////////////////////////////////////////////////////////////////////////////// Private #region 윈도우 열거하기 - EnumWindows(enumerateWindowDelegate, longParameter) /// <summary> /// 윈도우 열거하기 /// </summary> /// <param name="enumerateWindowDelegate">윈도우 열거하기 대리자</param> /// <param name="longParameter">LONG 매개 변수</param> /// <returns>처리 결과</returns> [DllImport("user32")] private static extern bool EnumWindows(EnumerateWindowDelegate enumerateWindowDelegate, IntPtr longParameter); #endregion #region 윈도우 표시 여부 구하기 - IsWindowVisible(windowHandle) /// <summary> /// 윈도우 표시 여부 구하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <returns>윈도우 표시 여부</returns> [DllImport("user32")] private static extern bool IsWindowVisible(IntPtr windowHandle); #endregion #region 윈도우 제목 구하기 - GetWindowText(windowHandle, stringBuilder, maximumCount) /// <summary> /// 윈도우 제목 구하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <param name="stringBuilder">문자열 빌더</param> /// <param name="maximumCount">최대 카운트</param> /// <returns>처리 결과</returns> [DllImport("user32", SetLastError = true)] private static extern int GetWindowText(IntPtr windowHandle, StringBuilder stringBuilder, int maximumCount); #endregion #region 윈도우 사각형 구하기 - GetWindowRect(windowHandle, rectangle) /// <summary> /// 윈도우 사각형 구하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <param name="rectangle">사각형</param> /// <returns>처리 결과</returns> [DllImport("user32")] private static extern bool GetWindowRect(IntPtr windowHandle, out RECT rectangle); #endregion #region 활성 윈도우 설정하기 - SetForegroundWindow(windowHandle) /// <summary> /// 활성 윈도우 설정하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <returns>처리 결과</returns> [DllImport("user32")] private static extern bool SetForegroundWindow(IntPtr windowHandle); #endregion #region 윈도우 스레드 프로세스 ID 구하기 - GetWindowThreadProcessId(windowHandle, processID) /// <summary> /// 윈도우 스레드 프로세스 ID 구하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <param name="processID">프로세스 ID</param> /// <returns>처리 결과</returns> [DllImport("user32")] private static extern uint GetWindowThreadProcessId(IntPtr windowHandle, out uint processID); #endregion #region 윈도우 최소화 여부 구하기 - IsIconic(windowHandle) /// <summary> /// 윈도우 최소화 여부 구하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <returns>윈도우 최소화 여부</returns> [DllImport("user32")] [return: MarshalAs(UnmanagedType.Bool)] private static extern bool IsIconic(IntPtr windowHandle); #endregion #region 윈도우 표시하기 - ShowWindow(windowHandle, showCommand) /// <summary> /// 윈도우 표시하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <param name="showCommand">표시 명령</param> /// <returns>처리 결과</returns> [DllImport("user32")] private static extern bool ShowWindow(IntPtr windowHandle, int showCommand); #endregion #region 전경 윈도우 구하기 - GetForegroundWindow() /// <summary> /// 전경 윈도우 구하기 /// </summary> /// <returns>전경 윈도우 핸들</returns> [DllImport("user32")] private static extern IntPtr GetForegroundWindow(); #endregion //////////////////////////////////////////////////////////////////////////////////////////////////// Field ////////////////////////////////////////////////////////////////////////////////////////// Private #region Field /// <summary> /// SW_MAXIMIZE /// </summary> private const int SW_MAXIMIZE = 3; #endregion //////////////////////////////////////////////////////////////////////////////////////////////////// Method ////////////////////////////////////////////////////////////////////////////////////////// Static //////////////////////////////////////////////////////////////////////////////// Public #region 크롬 브라우저 윈도우 정보 리스트 구하기 - GetChromeBrowserWindowInfoList() /// <summary> /// 크롬 브라우저 윈도우 정보 리스트 구하기 /// </summary> /// <returns></returns> public static List<WindowInformation> GetChromeBrowserWindowInfoList() { List<WindowInformation> windowInformationList = new List<WindowInformation>(); EnumWindows ( delegate(IntPtr windowHandle, IntPtr longParameter) { if(IsWindowVisible(windowHandle)) { uint processID; GetWindowThreadProcessId(windowHandle, out processID); try { Process process = Process.GetProcessById((int)processID); if(process.ProcessName.ToLower().Contains("chrome")) { StringBuilder stringBuilder = new StringBuilder(256); GetWindowText(windowHandle, stringBuilder, 256); GetWindowRect(windowHandle, out RECT rectangle); int width = rectangle.Right - rectangle.Left; int height = rectangle.Bottom - rectangle.Top; windowInformationList.Add ( new WindowInformation { WindowHandle = windowHandle, Title = stringBuilder.ToString(), ProcessID = processID, Width = width, Height = height } ); } } catch(ArgumentException) { } } return true; }, IntPtr.Zero ); return windowInformationList; } #endregion #region 메인 크롬 브라우저 윈도우 정보 구하기 - GetMainChromeBrowserWindowInformation(windowInformationList) /// <summary> /// 메인 크롬 브라우저 윈도우 정보 구하기 /// </summary> /// <param name="windowInformationList">윈도우 정보 리스트</param> /// <returns>윈도우 정보</returns> public static WindowInformation GetMainChromeBrowserWindowInformation(List<WindowInformation> windowInformationList) { if(windowInformationList == null || windowInformationList.Count == 0) { return null; } WindowInformation mainWindowInformation = null; int maximumArea = 0; foreach(WindowInformation windowInformation in windowInformationList) { int area = windowInformation.Width * windowInformation.Height; if(area > maximumArea) { maximumArea = area; mainWindowInformation = windowInformation; } } return mainWindowInformation; } #endregion #region 윈도우 활성화 대기하기 - WaitForWindowActivation(targetWindowHandle, timeout) /// <summary> /// 윈도우 활성화 대기하기 /// </summary> /// <param name="targetWindowHandle">타겟 윈도우 핸들</param> /// <param name="timeout">타임아웃 (단위 : 초)</param> public static void WaitForWindowActivation(IntPtr targetWindowHandle, int timeout = 30) { var startTime = DateTime.Now; while((DateTime.Now - startTime).TotalSeconds < timeout) { IntPtr foregroundWindow = GetForegroundWindow(); if(foregroundWindow == targetWindowHandle) { return; } Thread.Sleep(100); } throw new TimeoutException("지정 시간 내에 윈도우를 활성화하는데 실패했습니다."); } #endregion #region 활성탭 텍스트 구하기 - GetActiveTabText(windowInformation) /// <summary> /// 활성탭 텍스트 구하기 /// </summary> /// <param name="windowInformation">윈도우 정보</param> /// <returns>텍스트</returns> public static string GetActiveTabText(WindowInformation windowInformation) { IntPtr windowHandle = windowInformation.WindowHandle; if(IsIconic(windowHandle)) { ShowWindow(windowHandle, SW_MAXIMIZE); } SetForegroundWindow(windowHandle); WaitForWindowActivation(windowHandle); MessageHelper.ClickMouse(windowHandle, 1, 150); System.Windows.Forms.SendKeys.SendWait("^{a}"); Thread.Sleep(500); System.Windows.Forms.SendKeys.SendWait("^{c}"); Thread.Sleep(100); string text = Clipboard.GetText(); Thread.Sleep(100); MessageHelper.ClickMouse(windowHandle, 0, 200); return text; } #endregion } |
▶ MessageHelper.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 |
using System; using System.Runtime.InteropServices; using System.Threading; using System.Windows; namespace TestProject; /// <summary> /// 메시지 헬퍼 /// </summary> public static class MessageHelper { //////////////////////////////////////////////////////////////////////////////////////////////////// Import ////////////////////////////////////////////////////////////////////////////////////////// Static //////////////////////////////////////////////////////////////////////////////// Private #region 활성 윈도우 설정하기 - SetForegroundWindow(windowHandle) /// <summary> /// 활성 윈도우 설정하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <returns>처리 결과</returns> [DllImport("user32", SetLastError = true)] private static extern bool SetForegroundWindow(IntPtr windowHandle); #endregion #region 키보드 이벤트 발생시키기 - keybd_event(virtualKey, scanCode, flag, extraInformation) /// <summary> /// 키보드 이벤트 발생시키기 /// </summary> /// <param name="virtualKey">가상 키</param> /// <param name="scanCode">스캔 코드</param> /// <param name="flag">플래그</param> /// <param name="extraInformation">부가 정보</param> [DllImport("user32")] private static extern void keybd_event(byte virtualKey, byte scanCode, uint flag, uint extraInformation); #endregion #region 메시지 게시하기 - PostMessage(windowHandle, message, wordParameter, longParameter) /// <summary> /// 메시지 게시하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <param name="message">메시지</param> /// <param name="wordParameter">WORD 매개 변수</param> /// <param name="longParameter">LONG 매개 변수</param> /// <returns>처리 결과</returns> [DllImport("user32", SetLastError = true)] private static extern bool PostMessage(IntPtr windowHandle, uint message, IntPtr wordParameter, IntPtr longParameter); #endregion //////////////////////////////////////////////////////////////////////////////////////////////////// Field ////////////////////////////////////////////////////////////////////////////////////////// Private #region Field /// <summary> /// VK_CONTROL /// </summary> private const byte VK_CONTROL = 0x11; /// <summary> /// KEYEVENTF_KEYUP /// </summary> private const int KEYEVENTF_KEYUP = 0x0002; /// <summary> /// VK_U /// </summary> private const byte VK_U = 0x55; /// <summary> /// VK_A /// </summary> private const byte VK_A = 0x41; /// <summary> /// VK_C /// </summary> private const byte VK_C = 0x43; /// <summary> /// VK_W /// </summary> private const byte VK_W = 0x57; /// <summary> /// WM_LBUTTONDOWN /// </summary> private const uint WM_LBUTTONDOWN = 0x0201; /// <summary> /// WM_LBUTTONUP /// </summary> private const uint WM_LBUTTONUP = 0x0202; #endregion //////////////////////////////////////////////////////////////////////////////////////////////////// Method ////////////////////////////////////////////////////////////////////////////////////////// Static //////////////////////////////////////////////////////////////////////////////// Public #region 마우스 클릭하기 - ClickMouse(windowHandle, x, y) /// <summary> /// 마우스 클릭하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <param name="x">X</param> /// <param name="y">Y</param> public static void ClickMouse(IntPtr windowHandle, int x, int y) { PostMessage(windowHandle, WM_LBUTTONDOWN, IntPtr.Zero, MakeLongParameter(x, y)); Thread.Sleep(50); PostMessage(windowHandle, WM_LBUTTONUP, IntPtr.Zero, MakeLongParameter(x, y)); } #endregion #region 텍스트 구하기 - GetText(windowHandle) /// <summary> /// 텍스트 구하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <returns>텍스트</returns> public static string GetText(IntPtr windowHandle) { if(windowHandle != IntPtr.Zero) { SetForegroundWindow(windowHandle); // CTRL + A 키 메시지를 보낸다. SendCTRLKeyCombination(VK_A); Thread.Sleep(500); // CTRL + C 키 메시지를 보낸다. SendCTRLKeyCombination(VK_C); Thread.Sleep(100); ClickMouse(windowHandle, 1, 150); string text = Clipboard.GetText(); return text; } else { return null; } } #endregion //////////////////////////////////////////////////////////////////////////////// Private #region CTRL 키 조합 보내기 - SendCTRLKeyCombination(virtualKey) /// <summary> /// CTRL 키 조합 보내기 /// </summary> /// <param name="virtualKey">가상 키</param> private static void SendCTRLKeyCombination(byte virtualKey) { keybd_event(VK_CONTROL, 0, 0, 0); keybd_event(virtualKey, 0, 0, 0); keybd_event(virtualKey, 0, KEYEVENTF_KEYUP, 0); keybd_event(VK_CONTROL, 0, KEYEVENTF_KEYUP, 0); } #endregion #region LONG 매개 변수 만들기 - MakeLongParameter(lowValue, highValue) /// <summary> /// LONG 매개 변수 만들기 /// </summary> /// <param name="lowValue">하위 값</param> /// <param name="highValue">상위 값</param> /// <returns>LONG 매개 변수</returns> private static IntPtr MakeLongParameter(int lowValue, int highValue) { return (IntPtr)((highValue << 16) | (lowValue & 0xffff)); } #endregion } |
▶ WindowHelper.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
using System; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Interop; namespace TestProject; /// <summary> /// 윈도우 헬퍼 /// </summary> public static class WindowHelper { //////////////////////////////////////////////////////////////////////////////////////////////////// Import ////////////////////////////////////////////////////////////////////////////////////////// Static //////////////////////////////////////////////////////////////////////////////// Private #region 활성 윈도우 설정하기 - SetForegroundWindow(windowHandle) /// <summary> /// 활성 윈도우 설정하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <returns>처리 결과</returns> [DllImport("user32")] private static extern bool SetForegroundWindow(IntPtr windowHandle); #endregion #region 윈도우 표시하기 - ShowWindow(windowHandle, command) /// <summary> /// 윈도우 표시하기 /// </summary> /// <param name="windowHandle">윈도우 핸들</param> /// <param name="command">명령</param> /// <returns>처리 결과</returns> [DllImport("user32")] private static extern bool ShowWindow(IntPtr windowHandle, int command); #endregion //////////////////////////////////////////////////////////////////////////////////////////////////// Field ////////////////////////////////////////////////////////////////////////////////////////// Private #region Field /// <summary> /// SW_SHOW /// </summary> private const int SW_SHOW = 5; #endregion //////////////////////////////////////////////////////////////////////////////////////////////////// Method ////////////////////////////////////////////////////////////////////////////////////////// Static //////////////////////////////////////////////////////////////////////////////// Public #region 윈도우 앞으로 가져오기 - BringToFront(window) /// <summary> /// 윈도우 앞으로 가져오기 /// </summary> /// <param name="window">윈도우</param> public static void BringToFront(Window window) { WindowInteropHelper windowInteropHelper = new WindowInteropHelper(window); nint windowHandle = windowInteropHelper.Handle; ShowWindow(windowHandle, SW_SHOW); SetForegroundWindow(windowHandle); } #endregion } |
▶ WindowInformation.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
using System; namespace TestProject; /// <summary> /// 윈도우 정보 /// </summary> public class WindowInformation { //////////////////////////////////////////////////////////////////////////////////////////////////// Property ////////////////////////////////////////////////////////////////////////////////////////// Public #region 윈도우 핸들 - WindowHandle /// <summary> /// 윈도우 핸들 /// </summary> public IntPtr WindowHandle { get; set; } #endregion #region 제목 - Title /// <summary> /// 제목 /// </summary> public string Title { get; set; } #endregion #region 프로세스 ID - ProcessID /// <summary> /// 프로세스 ID /// </summary> public uint ProcessID { get; set; } #endregion #region 너비 - Width /// <summary> /// 너비 /// </summary> public int Width { get; set; } #endregion #region 높이 - Height /// <summary> /// 높이 /// </summary> public int Height { get; set; } #endregion } |