[C#/WPF] EventSetter 엘리먼트 : Event/Handler 속성을 사용해 ListBoxItem 객체를 마우드 더블 클릭시 처리하기
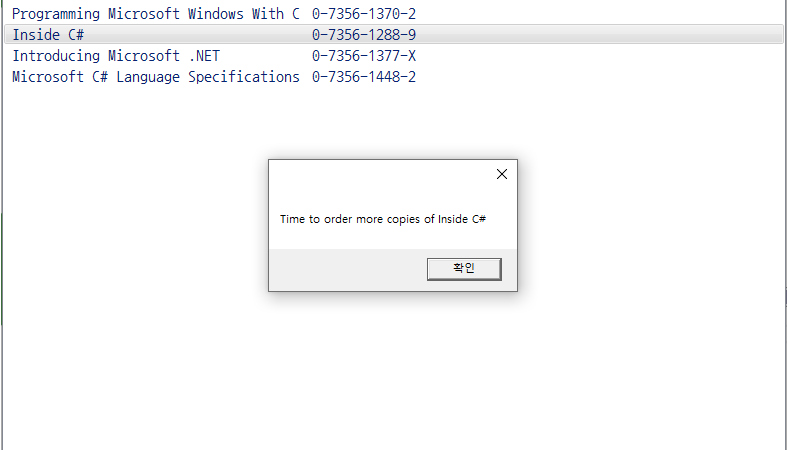
■ EventSetter 엘리먼트의 Event/Handler 속성을 사용해 ListBoxItem 객체를 마우드 더블 클릭시 처리하는 방법을 보여준다. ▶ MainWindow.xaml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 |
<Window x:Class="TestProject.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Width="800" Height="600" Title="TestProject" FontFamily="나눔고딕코딩" FontSize="16"> <Window.Resources> <XmlDataProvider x:Key="XmlDataProviderKey" XPath="Books"> <x:XData> <Books xmlns=""> <Book ISBN="0-7356-0562-9" Stock="in" Number="9"> <Title>XML in Action</Title> <Summary>XML Web Technology</Summary> </Book> <Book ISBN="0-7356-1370-2" Stock="in" Number="8"> <Title>Programming Microsoft Windows With C#</Title> <Summary>C# Programming using the .NET Framework</Summary> </Book> <Book ISBN="0-7356-1288-9" Stock="out" Number="7"> <Title>Inside C#</Title> <Summary>C# Language Programming</Summary> </Book> <Book ISBN="0-7356-1377-X" Stock="in" Number="5"> <Title>Introducing Microsoft .NET</Title> <Summary>Overview of .NET Technology</Summary> </Book> <Book ISBN="0-7356-1448-2" Stock="out" Number="4"> <Title>Microsoft C# Language Specifications</Title> <Summary>The C# language definition</Summary> </Book> </Books> </x:XData> </XmlDataProvider> <Style TargetType="ListViewItem"> <EventSetter Event="MouseDoubleClick" Handler="listViewItem_MouseDoubleClick" /> </Style> </Window.Resources> <ListView ItemsSource="{Binding Source={StaticResource XmlDataProviderKey}, XPath=Book}"> <ListView.View> <GridView> <GridViewColumn Width="300" Header="Title" DisplayMemberBinding="{Binding XPath=Title}" /> <GridViewColumn Width="150" Header="ISBN" DisplayMemberBinding="{Binding XPath=@ISBN}" /> </GridView> </ListView.View> </ListView> </Window> |
▶ MainWindow.xaml.cs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
using System.Windows; using System.Windows.Controls; using System.Windows.Input; using System.Xml; namespace TestProject { /// <summary> /// 메인 윈도우 /// </summary> public partial class MainWindow : Window { //////////////////////////////////////////////////////////////////////////////////////////////////// Constructor ////////////////////////////////////////////////////////////////////////////////////////// Public #region 생성자 - MainWindow() /// <summary> /// 생성자 /// </summary> public MainWindow() { InitializeComponent(); } #endregion //////////////////////////////////////////////////////////////////////////////////////////////////// Method ////////////////////////////////////////////////////////////////////////////////////////// Private #region 리스트 뷰 항목 마우스 더블 클릭시 처리하기 - listViewItem_MouseDoubleClick(sender, e) /// <summary> /// 리스트 뷰 항목 마우스 더블 클릭시 처리하기 /// </summary> /// <param name="sender">이벤트 발생자</param> /// <param name="e">이벤트 인자</param> private void listViewItem_MouseDoubleClick(object sender, MouseButtonEventArgs e) { XmlElement bookXmlElement = (sender as ListViewItem).Content as XmlElement; if(bookXmlElement == null) { return; } if(bookXmlElement.GetAttribute("Stock") == "out") { MessageBox.Show("Time to order more copies of " + bookXmlElement["Title"].InnerText); } else { MessageBox.Show(bookXmlElement["Title"].InnerText + " is available."); } } #endregion } } |
TestProject.zip